Integrating the Widget
The checkout widget is a payment interface that enables you to securely collect payments from customers on your website or application. With various payment options like credit cards, UPI, and net banking, the widget ensures a smooth checkout experience for your customers.
Here’s an outline of the end-to-end payment process through Zoho Payments' checkout widget.
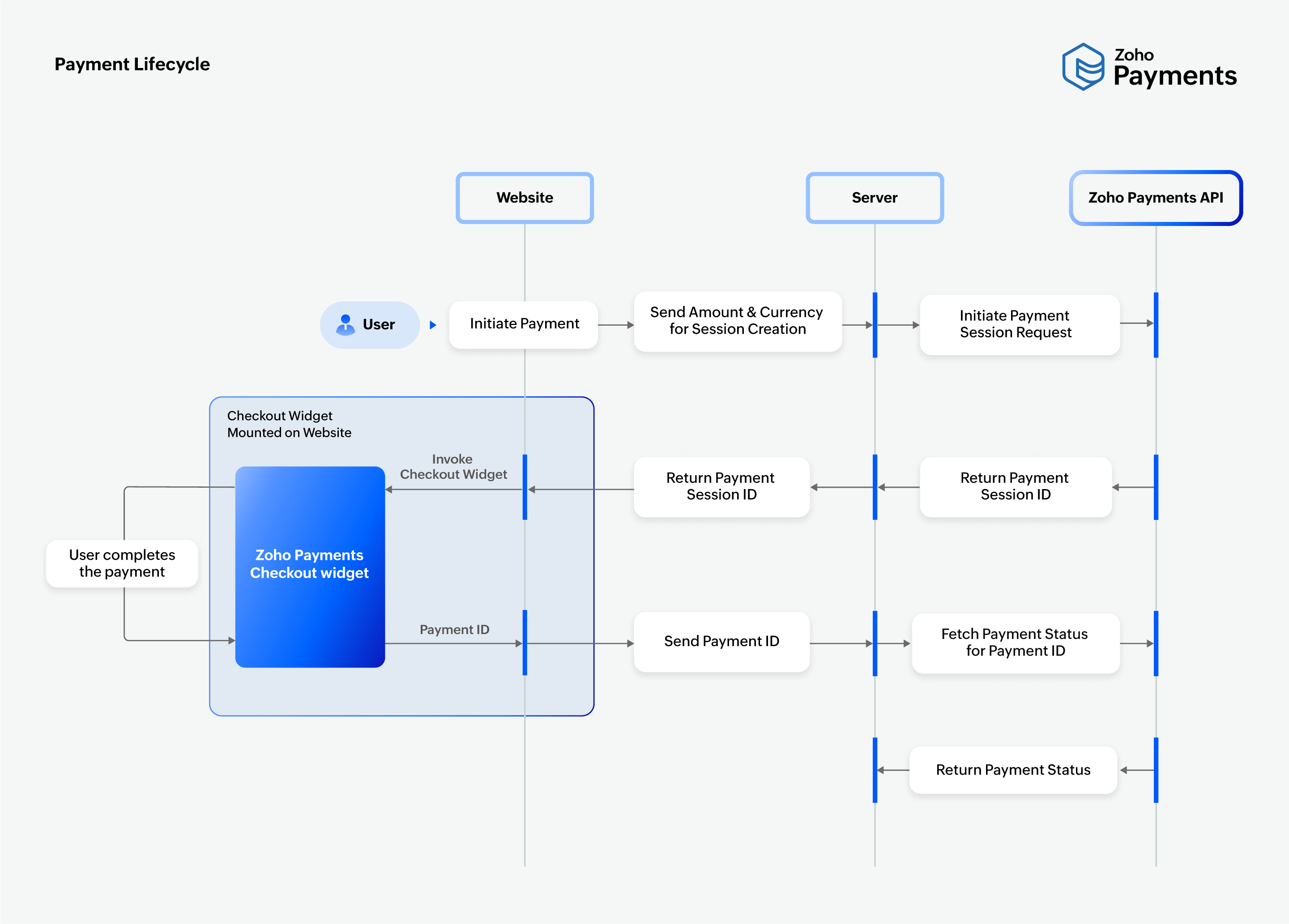
Follow the steps below to configure the checkout widget on your website.
1. Establish Communication
To communicate with our APIs and webhooks, you must first establish connectivity. Ensure that your setup allows or whitelists the Zoho Payments domain, payments.zoho.in.
2. Create Payment Session
To initiate the payment process, you need to create a payment session on your server by calling the Payment Session Create API. Ensure you follow the steps below to obtain the payment_session_id:
- Collect payment information from the website (amount, currency, etc.).
- Call the Payment Session Create API using the OAuth token generated from your server.
- The API will return a
payment_session_id.
Use this payment_session_id
while integrating the checkout widget.
3. Integrate Checkout Widget
After receiving the payment_session_id
from your server, you can invoke the checkout widget on your website. Zoho Payments' script helps you manage the payment flow easily.
- Add the Zoho Payments script given below to your website or application.
<script src="https://static.zohocdn.com/zpay/zpay-js/v1/zpayments.js"></script>
- Initialise the script using the API key generated from Zoho Payments' Developers Space.
let config = {
"account_id": "23137556",
"domain": "IN",
"otherOptions": {
"api_key": "1000.41d9xxxxxxxxxxxxxxxxxxxxxxxxc2d1.8fccxxxxxxxxxxxxxxxxxxxxxxxx125f"
}
}
let instance = new window.ZPayments(config);
- Invoke the
requestPaymentMethod()
function withpayment_session_id
to initiate the payment.
try {
let options = {
"amount": "100.5",
"currency_code": "INR",
"payments_session_id": "2000000012001",
"currency_symbol": "₹",
"business": "Zylker",
"description": "Purchase of Zylker electronics.",
"address": {
"name": "Canon",
"email": "canonbolt@zylker.com"
}
};
let data = await instance.requestPaymentMethod(options);
} catch (err) {
if (err.code != "widget_closed") {
// Handle Error
}
} finally {
await instance.close();
}
Once the checkout widget is integrated, you can collect payments, gather transaction details such as amount, currency, and customer information, and verify the payment status. You verify the payment status using the payment_id
provided after the user completes the payment.
4. Confirm Payment
Once the customer completes the payment, you can confirm the payment using the requestPaymentMethod()
function. This function will return a response that includes the payment_id.
This ID can be used to track the payment status. If a payment fails due to issues like payment errors or user cancellations, you can implement error handling.
5. Verify Payment Status
After receiving the payment_id,
the payment status can be verified using the Payment Retrieve API. This ensures that the payment has been completed successfully. Based on the status returned (success, failure, or pending), update your system.
Alternatively, you can configure webhooks to receive the events payment.success and payment.failed to receive the final payment status update to your server directly.